Why complicate simplicity?
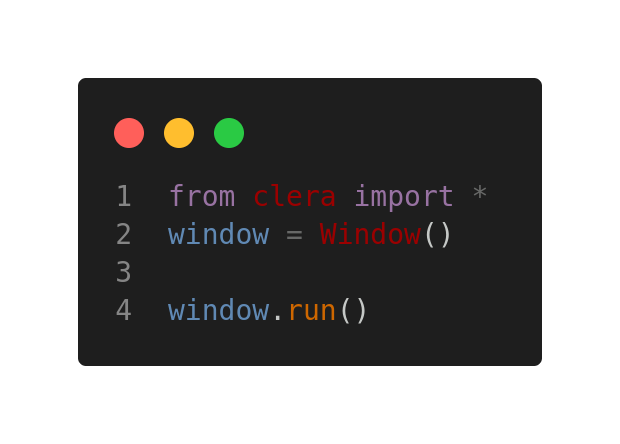
Clera is python module for developing GUI quickly and simply with low learning curve
CORE OF CLERA
- Simplicity
- Flexibility
- Quality
- Speed
- Windows
- Linux
- Mac
Why complicate simplicity?
Clera is python module for developing GUI quickly and simply with low learning curve
CORE OF CLERA
DOCUMENTATION
Clera is python module for writing Graphical User Interfaces and it is developed on PySide6 module. This means using clera, you can write GUI applications for all platforms (Windows, Linux, Mac and others) with native interface.
Clera is easy to learn, fast to write and made as simple as possible due to its motto: “Why Complicate SImplicity?”, this makes it best choice for anyone who wants to develop a GUI application without much hussle and those who wants to try GUI development in general. Clera is pronounced “Clearer” which represents “more clear” based on the idea of writing great GUI with fewer lines of clear code.
In order to use clera you need to have basic knowlege of python. If you have no knowlege in python, I recommend you learn a little about python and its basics. Since clera is written with python, its fundermental usage is based on python and this will come later when writing the core functionality of widgets and elements of your GUI application.
NOTE: Clera has a lot of classes and you don't need to learn all of them. All you need to learn are the classes you will use by recognizing what you want and need.
Setting The Foundation
What is a Window()?
A window is a viewing area on a computer display screen that allows viewing areas as part of a Graphical User Interface (GUI). Hence the Window() class is the most important part of clera because is the class on which all other this such as widget, element and layouts are drawn. Without the “Window()” class, there is will be no “window”. You can create window by importing it from the clera module “from clera import Window”, calling the “Window()” class and to display the window, call the "run()" method on it.
NOTE: It is recommended to import every widget, layout and elemnts in clera individually.
EXAMPLE
1 | from clera import Window | 📋 |
2 | Window().run() |
...and you've created your first window in clera. When using clera, it is recommended to assign the “Window()” class to a variable “window” and calling the run on it. All elements of the window goes in between them.
EXAMPLE
1 | from clera import Window | 📋 |
2 | window = Window() # initialize window | |
3 | # All elements of window goes here! | |
4 | window.run() # run window |
The Window Contents
A blank window is not quite useful hence adding of widgets and layouts. We can consider widgets as books that we are arranging of a book shelf (layouts). These books are arranged in rows from top to bottom. That is the widget and layout system clera uses.
Nested list of which the main list signifies the bookshelf and the list in it each signifies the rows in the bookshelf. The books in the rows of the bookshelf represents the widgets.
There are are two types of layouts in clera. These are the Box Layout and the Grid Layout..
Box Layout
Takes widgets in the nested array and initilize widget position and appearance in application window. Box Layout stretches widgets to occupy row if there is a row longer than its row.
Grid Layout - [Under Development]
Automatically arranges the widgets in grids. Takes the row of widget structure and puts it in grid.
1 takes the grid position (0, 0), 2 takes the grid position (0, 1), 3 takes the grid position (1,0) just like a matrix.
Quick Start
Welcome to clera! The next question is how to create a window with widgets. Here a simple hello clera application showing initialization of the window ant the layout theory.
1 | # Simple Hello Clera! Application | 📋 |
2 | from clera import * | |
3 | window = Window() # Initialize window | |
4 | ||
5 | # Setting up widgets structure and layout | |
6 | Box([ | |
7 | [Text('Hello Clera!', alignment=center)] | |
8 | ]) | |
9 | ||
10 | window.run() # Running the window |
You can do alot more with clera than just adding text on a window screen. Lets see a more complex example where on a button click, the window updates with Hello Clera.
1 | # Complex Hello Clera! Application | 📋 |
2 | from clera import * | |
3 | window = Window() # Initialize window | |
4 | ||
5 | # function to run when button is clicked | |
6 | def message(): | |
7 | text_widget = Text('Hello Clera!', alignment=center) | |
8 | GET('-button-').update(text_widget) | |
9 | ||
10 | ''' | |
11 | Adding the Button widget, with button text, | |
12 | function to run when it has been clicked and | |
13 | id to for reference in other parts of the code. | |
14 | ''' | |
15 | Box([ | |
16 | [Button('click me', message, id='-button-')] | |
17 | ]) | |
18 | ||
19 | window.run() # Running the window |